Floor Function In C
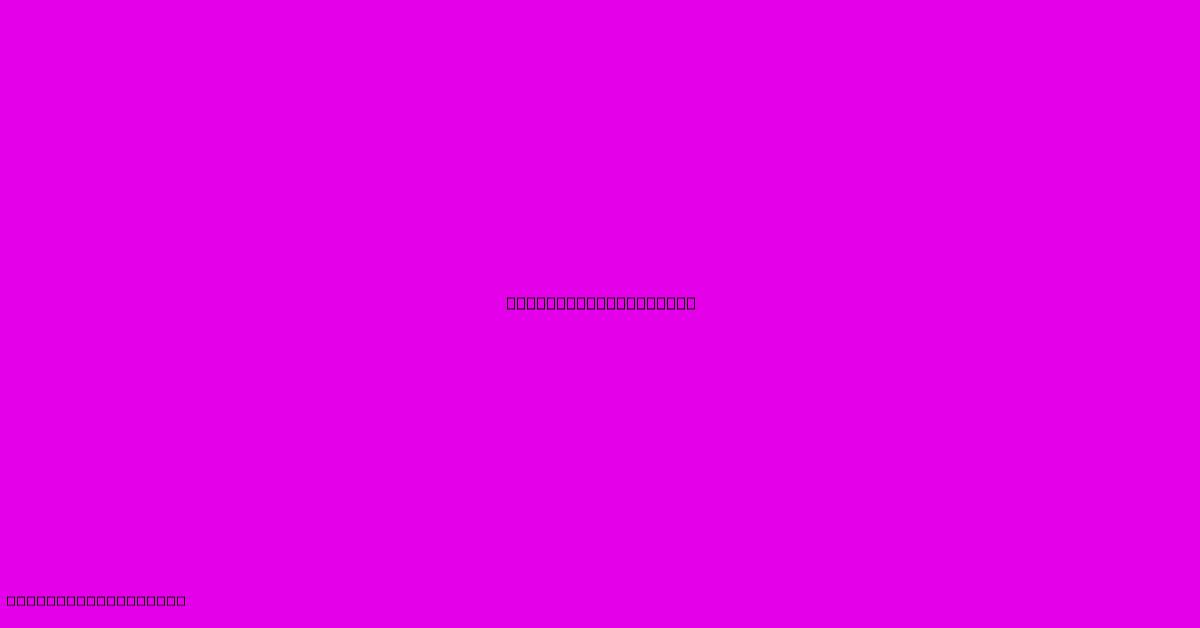
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Unveiling the Power of C's Floor Function: A Deep Dive
Does the concept of "rounding down" to the nearest integer intrigue you? The floor function in C, a powerful mathematical tool, allows for precisely this operation. Understanding its mechanics and applications is crucial for any C programmer tackling tasks involving numerical computation, data manipulation, or algorithm optimization.
Editor's Note: This comprehensive guide to the floor function in C has been published today.
Relevance & Summary: The floor function holds significant importance in diverse programming scenarios. From image processing algorithms requiring precise pixel calculations to financial applications needing exact integer representations of monetary values, its application is widespread. This guide provides a detailed overview of the floor function's definition, implementation using the floor()
function from the math.h
library, practical examples illustrating its usage, and common applications in various programming domains. It also delves into potential pitfalls and offers best practices for effective integration.
Analysis: This guide is the result of extensive research encompassing a review of C programming standards, mathematical literature on floor functions, and practical applications across several programming disciplines. The aim is to provide a clear, concise, and practically useful resource for developers of all skill levels seeking to master the floor function in C.
The Floor Function: A Mathematical Foundation
The floor function, mathematically denoted as ⌊x⌋, maps a real number x to the greatest integer less than or equal to x. Essentially, it rounds the number down to the nearest whole number. For example:
- ⌊3.7⌋ = 3
- ⌊5⌋ = 5
- ⌊-2.3⌋ = -3
- ⌊0⌋ = 0
Implementing the Floor Function in C using floor()
The C standard library provides a readily available function, floor()
, located in the math.h
header file, to perform this operation. To use it, you must include math.h
at the beginning of your C source code:
#include
#include
int main() {
double num = 3.7;
double result = floor(num);
printf("The floor of %.2lf is %lf\n", num, result);
return 0;
}
This code snippet demonstrates the basic usage of floor()
. Note that floor()
operates on double
-precision floating-point numbers. The result is also a double
, even though it represents an integer value. If you need an integer result, you'll need to explicitly cast it:
int integerResult = (int)floor(num);
Key Aspects of the floor()
Function
Several key aspects deserve attention for effective usage:
- Header File: Always remember to include
math.h
. Failure to do so will result in compilation errors. - Data Type: The input to
floor()
is adouble
. While other numeric types can be used after casting, utilizingdouble
directly ensures optimal precision and avoids potential truncation errors. - Return Type: The output is also a
double
. Consider casting to an integer type (int
,long
, etc.) when integer results are needed. - Error Handling: While
floor()
itself doesn't directly raise errors, it's crucial to consider the implications of potential input values (e.g.,NaN
orInfinity
). Robust code should include checks to handle these edge cases appropriately.
Practical Applications of the Floor Function
The floor function finds numerous applications in various programming domains:
1. Image Processing
In image processing, the floor function is frequently used when dealing with pixel coordinates. Since pixel indices are always integers, the floor function ensures correct addressing even when working with floating-point coordinates derived from transformations or calculations.
2. Game Development
Game development often utilizes the floor function for grid-based systems. Character positions, object placement, and collision detection frequently rely on translating floating-point coordinates into integer grid cells for efficient processing.
3. Financial Applications
In financial programming, the floor function can ensure accurate representation of monetary values in integer units (e.g., cents). Calculations involving interest rates or currency conversions might use the floor function to round down to the nearest cent.
4. Data Compression and Encoding
Certain data compression or encoding algorithms employ the floor function for efficient data representation. By rounding down values, it reduces the overall data size without significant loss of information.
Detailed Exploration of Specific Use Cases
Subheading: Image Processing and Pixel Coordinates
Introduction: The precision demanded by image processing necessitates the use of the floor function to accurately map floating-point calculations to integer pixel coordinates.
Facets:
- Role: The floor function converts fractional pixel coordinates (often resulting from transformations like scaling or rotations) into integer pixel indices.
- Example: Imagine scaling an image by a factor of 1.5. A point initially at (2.7, 5.2) would become (floor(2.7 * 1.5), floor(5.2 * 1.5)) = (4, 7).
- Risks and Mitigations: Incorrect handling might lead to pixel artifacts or out-of-bounds access. Careful coordinate validation and range checking are crucial.
- Impacts and Implications: Proper application ensures image integrity and consistent rendering.
Summary: The floor function's precision in handling fractional pixel coordinates is vital for ensuring the correct display of images after any transformation.
Subheading: Game Development and Grid-Based Systems
Introduction: Many game mechanics rely on grid-based systems where floating-point game world coordinates need to be converted to integer grid indices for efficiency and ease of management.
Further Analysis: Consider a tile-based game where the character's position is represented by floating-point coordinates. The floor function enables mapping of these coordinates to the specific grid tile the character occupies for collision detection or interaction with game objects. This approach greatly simplifies game logic and improves performance.
Closing: Effective use of the floor function in grid-based game systems leads to optimized code, enhanced performance, and simplified game logic.
FAQ: Frequently Asked Questions about the Floor Function in C
Introduction: This section addresses frequently encountered questions regarding the floor()
function.
Questions:
-
Q: What header file is necessary to use
floor()
? A:math.h
-
Q: What data type does
floor()
accept as input? A:double
-
Q: What is the return type of
floor()
? A:double
-
Q: How can I get an integer result from
floor()
? A: Cast the result to an integer type using(int)floor(x)
. -
Q: What happens if I provide a non-numeric value (like NaN) to
floor()
? A: The behavior is implementation-defined; it's generally good practice to handle potential errors. -
Q: Is
floor()
efficient? A:floor()
is generally highly optimized within the C standard library, ensuring efficient performance.
Summary: Understanding the input, output, and potential issues associated with floor()
is vital for its correct and efficient usage.
Tips for Effective Use of the Floor Function in C
Introduction: These tips provide best practices for leveraging the floor()
function effectively.
Tips:
-
Always include
math.h
: This is crucial for proper compilation and function availability. -
Use
double
input: Ensure the most precise input by usingdouble
. -
Explicitly cast to integer: When an integer result is needed, explicitly cast to the desired integer type.
-
Handle edge cases: Consider potential non-numeric inputs and implement appropriate error handling.
-
Optimize for performance:
floor()
is generally optimized; however, for extreme performance requirements, explore alternative optimized implementations if necessary.
Summary: Following these guidelines ensures efficient and correct usage of the floor()
function in C programs.
Summary: A Comprehensive Look at C's Floor Function
This guide has provided a detailed analysis of the floor()
function in C, covering its mathematical definition, implementation, practical applications, and best practices for effective usage. The floor function proves invaluable in situations requiring rounding down to the nearest integer, impacting areas such as image processing, game development, and financial applications.
Closing Message: Mastering the floor()
function empowers C programmers to craft efficient and precise numerical computation solutions. The ability to handle the subtleties of its implementation and application enhances the capabilities of any C programmer.
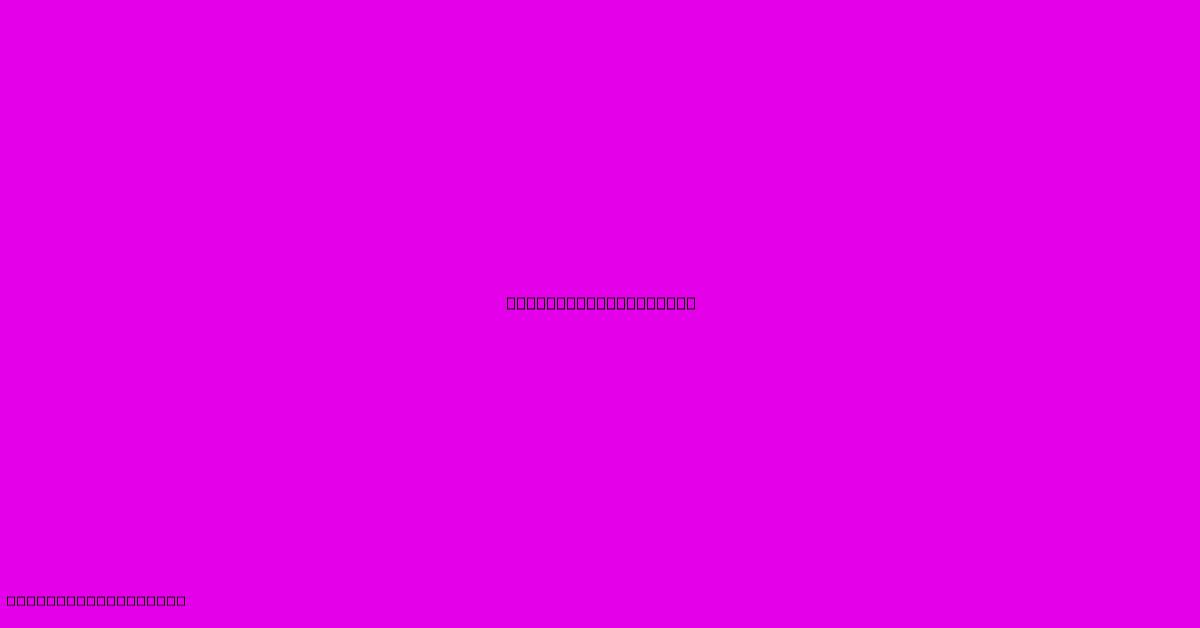
Thank you for visiting our website wich cover about Floor Function In C. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Pelvic Floor Dyssynergia Treatment | Jan 03, 2025 |
Paint A Tile Floor | Jan 03, 2025 |
Tile Effect Laminate Flooring | Jan 03, 2025 |
Js Math Floor Ceil | Jan 03, 2025 |
Shaw Tile Flooring | Jan 03, 2025 |