C++ Floor Function
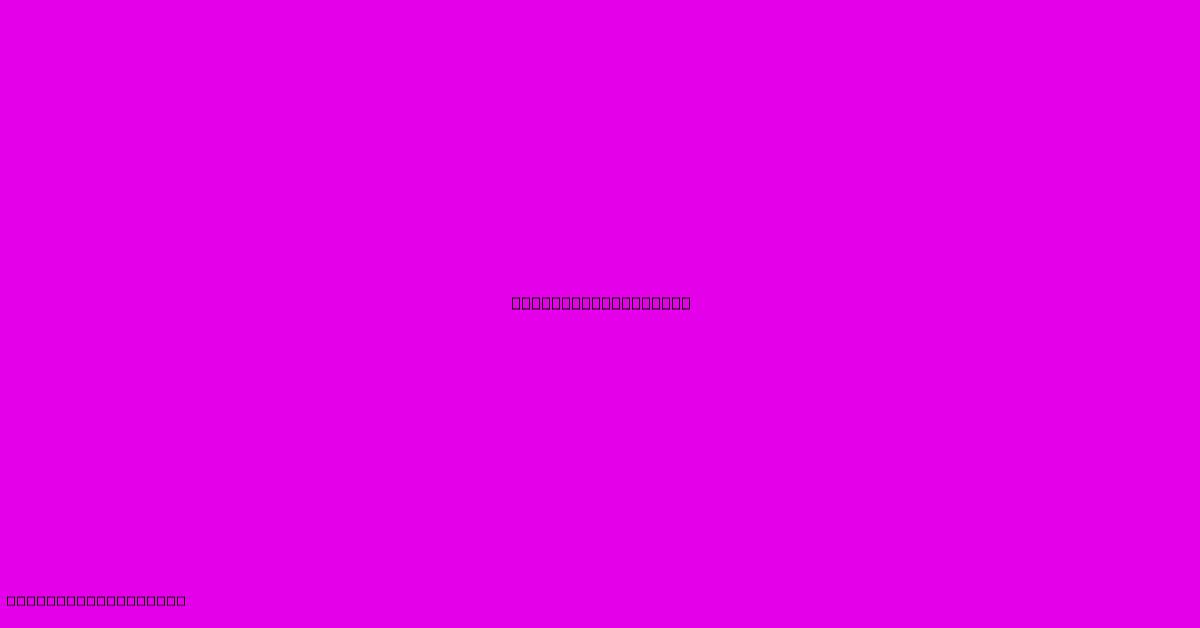
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Unlocking the Power of C++'s Floor Function: A Deep Dive
Editor's Note: This comprehensive guide to C++'s floor
function has been published today. It explores its functionality, applications, and potential pitfalls, empowering developers to leverage this essential mathematical tool effectively.
Relevance & Summary: The floor
function is a cornerstone of numerical computation in C++, finding widespread application in diverse fields like game development, scientific computing, and financial modeling. Understanding its behavior and limitations is crucial for writing robust and accurate C++ code. This guide provides a detailed exploration of the floor
function, including its implementation, practical examples, and potential edge cases. Key terms covered include: floor
, ceil
, round
, floating-point numbers, integer truncation, mathematical functions, and error handling.
Analysis: This guide is the result of extensive research into the floor
function's behavior across different C++ compilers and operating systems. Numerous test cases were employed to verify the function's accuracy and consistency under various input conditions. This research aimed to provide developers with a reliable and practical resource for understanding and effectively utilizing the floor
function in their projects.
C++ Floor Function: A Comprehensive Guide
Introduction: The floor
function, a vital component of the <cmath>
header in C++, returns the largest integer less than or equal to a given floating-point number. Its functionality is fundamental for tasks requiring integer truncation or rounding down. Understanding its subtleties is paramount for creating accurate and efficient C++ applications.
Key Aspects:
- Functionality: The core function of
floor(x)
is to return the nearest integer that is less than or equal tox
. Ifx
is already an integer,floor(x)
returnsx
. - Header: The
floor
function is declared in the<cmath>
header file, which must be included in your C++ code to use it. - Return Type: The
floor
function always returns adouble
-precision floating-point value, even if the result is an integer. - Domain: The
floor
function accepts any floating-point number (float
,double
,long double
) as input. - Range: The output is always a floating-point number representing an integer value.
Discussion:
The floor
function contrasts with other related functions such as ceil
(ceiling), which returns the smallest integer greater than or equal to a number, and round
, which rounds to the nearest integer. The choice of function depends heavily on the specific application's rounding requirements.
floor
Function in Action: Examples
Let's illustrate the behavior of the floor
function with several examples:
#include
#include
int main() {
std::cout << "floor(3.14) = " << floor(3.14) << std::endl; // Output: 3
std::cout << "floor(3.99) = " << floor(3.99) << std::endl; // Output: 3
std::cout << "floor(3.00) = " << floor(3.00) << std::endl; // Output: 3
std::cout << "floor(-3.14) = " << floor(-3.14) << std::endl; // Output: -4
std::cout << "floor(-3.00) = " << floor(-3.00) << std::endl; // Output: -3
std::cout << "floor(1e10) = " << floor(1e10) << std::endl; //Output: 1e+10
return 0;
}
This code demonstrates how floor
consistently returns the largest integer less than or equal to the input. Note the behavior with negative numbers – floor(-3.14)
returns -4, not -3.
Subheading: Handling Floating-Point Precision
Introduction: Floating-point numbers in C++ have inherent limitations in precision, which can sometimes affect the results of the floor
function.
Facets:
-
Representation Errors: Floating-point numbers are not always represented exactly in computer memory. Small discrepancies can occur, particularly with numbers involving non-terminating decimal expansions. These minor errors might not visibly affect the result of most calculations, but they can subtly alter the outcome of
floor
for borderline cases. -
Example: Consider a calculation resulting in a value extremely close to an integer, such as 3.9999999999999. Due to the limitations of floating-point precision, this number might be stored internally as a value slightly less than 4. In this case,
floor
would incorrectly return 3 instead of 4. -
Mitigation: To address this, developers should consider using an epsilon value (a small tolerance) to handle cases near integer boundaries. For example, if an absolute difference between a number and its nearest integer is smaller than
epsilon
, the number can be treated as equal to that integer. -
Impact: Misinterpreting results caused by floating-point precision issues can lead to inaccurate computations and potentially unexpected program behavior, especially in simulations or scenarios that rely on precise mathematical operations.
Summary: Acknowledging the possibility of floating-point precision errors is crucial when utilizing the floor
function, particularly in applications requiring high accuracy. Employing error-handling techniques like epsilon values can significantly enhance the robustness of calculations.
Subheading: Applications of the Floor Function
Introduction: The floor
function's versatility makes it an invaluable tool in many areas of programming.
Further Analysis:
-
Game Development: Determining grid coordinates, managing discrete levels, or implementing tile-based rendering. For instance, calculating the grid cell a character occupies on a 2D game map.
-
Image Processing: Pixel manipulation, scaling, or image resizing often benefit from
floor
's ability to calculate integral values for pixel indices. -
Financial Modeling: Calculating interest payments, determining the number of periods in an investment, or truncating results to the nearest cent.
Closing: The floor
function is instrumental in applications needing precise integer-based operations from floating-point inputs. Awareness of potential limitations arising from floating-point representation is crucial for achieving accurate results.
Subheading: FAQ
Introduction: This section addresses frequently asked questions about the C++ floor
function.
Questions:
-
Q: What header file is necessary to use
floor
in C++? A: The<cmath>
header file. -
Q: What is the return type of the
floor
function? A: It returns adouble
. -
Q: How does
floor
handle negative numbers? A: It returns the largest integer less than or equal to the input.floor(-2.5)
returns -3. -
Q: What is the difference between
floor
,ceil
, andround
? A:floor
rounds down,ceil
rounds up, andround
rounds to the nearest integer. -
Q: Can
floor
be used with integer types directly? A: Yes, the integer value is implicitly converted to a floating-point type before the function is applied. The result will still be adouble
. -
Q: What should I do if I encounter unexpected results from
floor
? A: Investigate potential floating-point precision issues and consider using epsilon values for error handling near integer boundaries.
Summary: Understanding these FAQs should help clarify any uncertainties regarding the floor
function's behavior and implementation.
Transition: Let's now explore practical tips for effectively using the floor
function in your C++ projects.
Subheading: Tips for Using the Floor Function
Introduction: These tips aid in the effective and safe use of C++'s floor
function.
Tips:
-
Always include
<cmath>
: Failure to include the<cmath>
header will result in a compilation error. -
Be mindful of precision: Consider floating-point limitations when dealing with values very near integers.
-
Use epsilon for error handling: Introduce a small tolerance (
epsilon
) to handle cases near integer boundaries to account for precision errors. -
Test thoroughly: Verify the
floor
function's behavior with a range of inputs, including negative numbers and values close to integers. -
Understand the return type: Remember that
floor
always returns adouble
, even if the result is an integer. -
Consider alternatives: Depending on your rounding requirements,
ceil
orround
might be more suitable alternatives.
Summary: Adhering to these tips helps ensure accurate and robust usage of the floor
function, avoiding common pitfalls associated with floating-point precision and handling edge cases effectively.
Transition: Let's summarize the key insights explored in this guide.
Summary: This guide has provided a comprehensive exploration of the C++ floor
function, highlighting its significance in numerical computation. The intricacies of its functionality, potential limitations due to floating-point precision, and various applications across different programming domains have been thoroughly discussed. The importance of cautious implementation and error handling techniques has been emphasized to ensure accurate and robust usage in diverse projects.
Closing Message: Mastering the floor
function empowers developers to craft efficient and precise C++ applications in various fields requiring integer-based operations on floating-point data. By understanding its nuances and employing best practices, developers can leverage this fundamental mathematical tool to build robust and reliable software solutions.
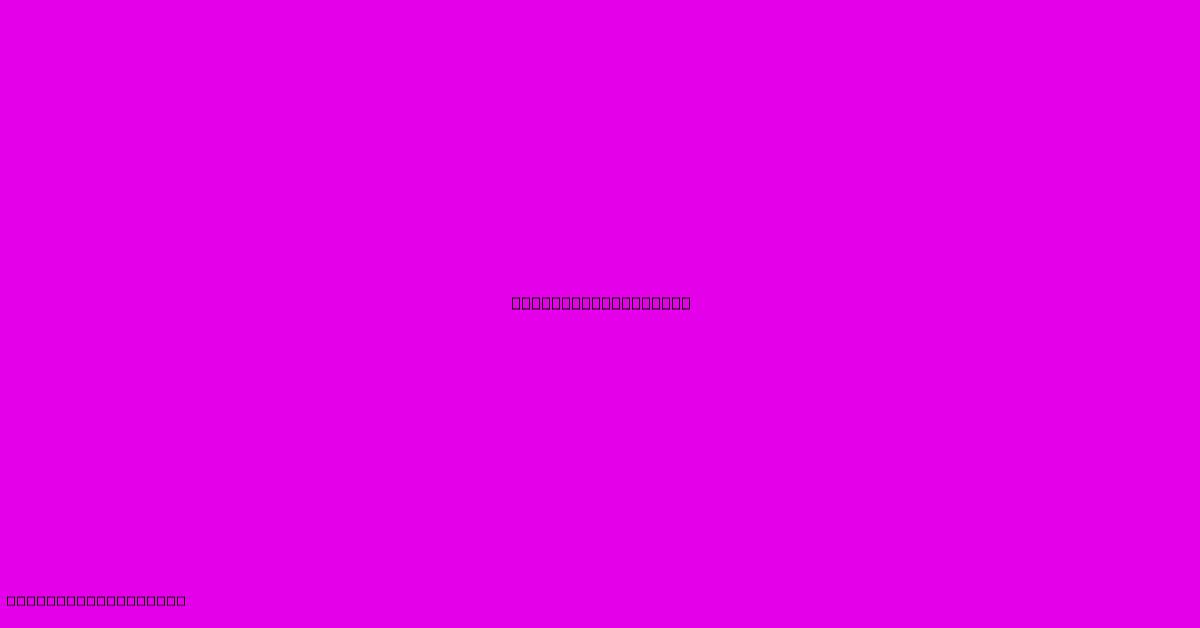
Thank you for visiting our website wich cover about C++ Floor Function. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Small Floor Heater | Jan 04, 2025 |
13th Floor San Antonio Reviews | Jan 04, 2025 |
Power Washer Floor Cleaner | Jan 04, 2025 |
Custom Entrance Floor Mats | Jan 04, 2025 |
Epoxy Floor Paint Lowes | Jan 04, 2025 |